Understanding Const Assertions in TypeScript: Benefits, Usage, and Best Practices
5 min read
TypeScript, a superset of JavaScript, provides powerful static typing features that enhance code quality and maintainability. One such feature is const assertions, which allow developers to declare values as immutable. In this blog post, we'll explore the concept of const assertions, understand how to use them effectively, and examine the advantages they offer to TypeScript developers.
What are Const Assertions?
Const assertions are a TypeScript feature introduced in version 3.4. They provide a way to tell the compiler that a value should not be widened to its inferred type. In other words, const assertions allow you to specify that a value is exactly what it appears to be and should not be modified later.
Using Const Assertions:
To use const assertions, you append the as const
assertion to a value or expression. For example:
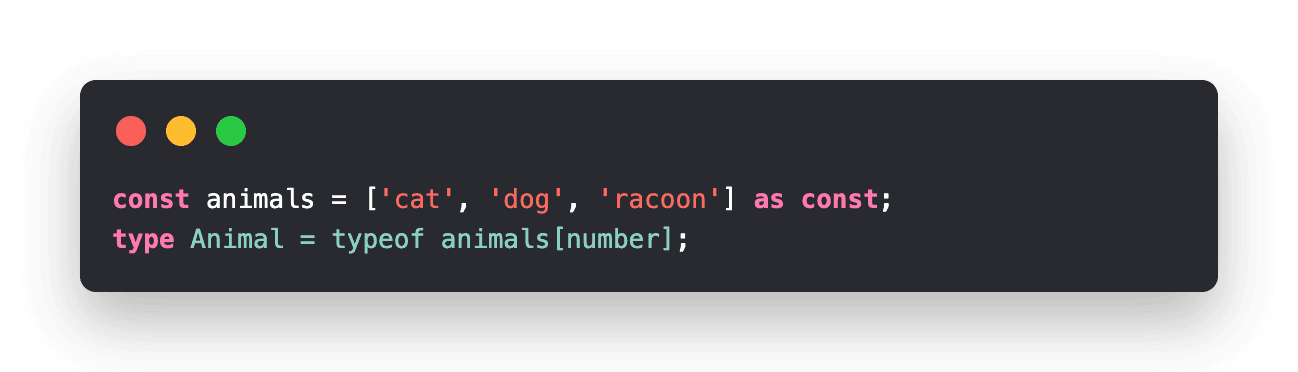
The animals array is declared and assigned with three string literals: cat
, dog
, and racoon
.
The as const
assertion is applied to the animals array. This assertion ensures that the inferred type of animals is not widened to string[]
(an array of strings) but remains a tuple of string literals, i.e., ['cat', 'dog', 'racoon']
as the exact type.
The typeof
operator is used in conjunction with the number index to create a type alias called Animal
. typeof animals
refers to the type of the animals array, which is ['cat', 'dog', 'racoon']
(a tuple of string literals). By accessing the number index, typeof animals[number]
represents the union of all possible values in the animals array, i.e., 'cat' | 'dog' | 'racoon'
.
Therefore, the Animal type alias represents a union type that can only have the values cat
, dog
, or racoon
. It provides a convenient way to define a type that is limited to a specific set of string literals.
Here's an example usage of the Animal type:
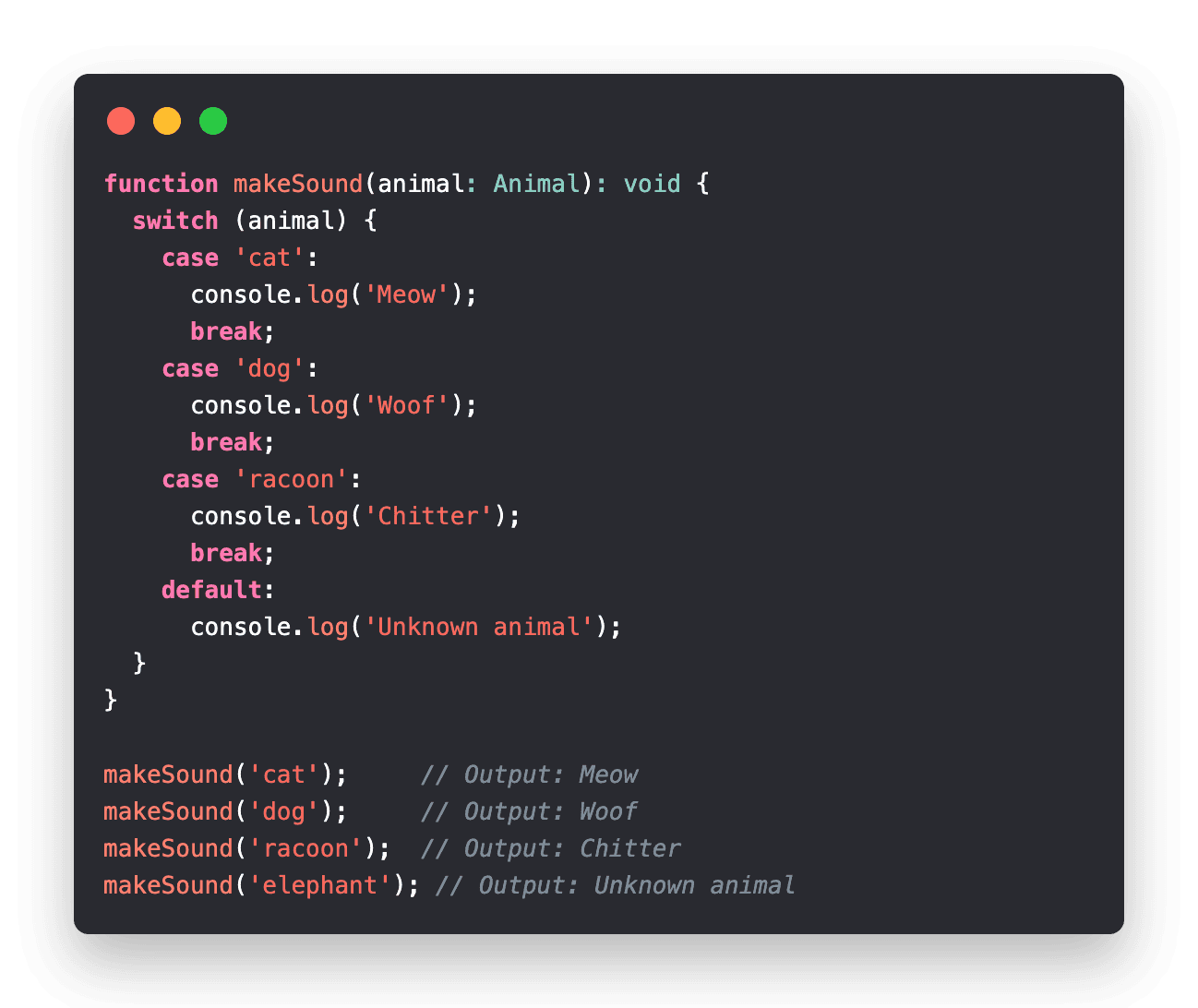
In the example usage, the makeSound
function accepts an argument of type Animal
, which means it can only accept one of the three specific string literals: cat
, dog
, or racoon
. This helps ensure type safety by preventing the accidental passing of invalid animal values.
Benefits of Const Assertions:
- Enhanced Type Safety: Const assertions provide stronger type checking by preventing unintentional modifications to values. This ensures that the values remain constant throughout the codebase, reducing the risk of bugs caused by accidental mutations.
- Improved Performance: When you use const assertions, TypeScript can optimize your code more effectively. The compiler knows that the values are immutable, allowing it to make certain assumptions and optimize the generated JavaScript accordingly.
- Narrowed Literal Types: Const assertions narrow down the inferred types of values to their exact literals. This enables the compiler to provide more precise type information and enables better autocompletion, static analysis, and documentation within your IDE.
- Better API Contracts: When creating libraries or interfaces, const assertions allow you to define exact types for certain properties or values. This provides a clear and concise contract, making it easier for consumers to understand how to interact with your code.
When to Use Const Assertions:
- String Literals: When working with string values that represent specific fixed values, such as status codes or configuration keys, const assertions ensure that the values remain constant and prevent unintended changes.
- Numeric Literals: Similar to string literals, const assertions help maintain the exact numeric values, such as fixed numeric IDs or constants used for calculations.
- Object Properties: When defining object properties, const assertions allow you to mark specific properties as readonly, ensuring that their values cannot be modified after initialization.
- Discriminated Union Types: Const assertions can be helpful when defining discriminated union types, where specific properties determine the possible values. Marking these properties as as const provides additional type safety and avoids potential issues.
Best Practices:
- Use const assertions sparingly: While const assertions offer benefits, it's important to use them judiciously. Applying const assertions to every value may hinder flexibility and the ability to refactor code in the future.
- Combine with
Readonly
andReadonlyArray
: When dealing with object properties or arrays, consider using Readonly<T> or ReadonlyArray<T> in conjunction with const assertions. This further enhances immutability and helps prevent accidental modifications. - Document Your Intent: Since const assertions affect the behavior of your code, it's important to document the intent behind using them. Clearly communicate to other developers that the values should not be modified.
Const assertions in TypeScript provide a powerful mechanism for enforcing immutability and strengthening type safety. By leveraging const assertions effectively, developers can write more robust and maintainable code.
Keep in touch and happy coding